Another thing the game has (and will build on in the next release) is unlockable character profiles with some information about each character, in addition to some bonus features unlocked from them. As far as things I’ve posted here go, this is a fairly straightforward one, but I figured I’d share anyway.
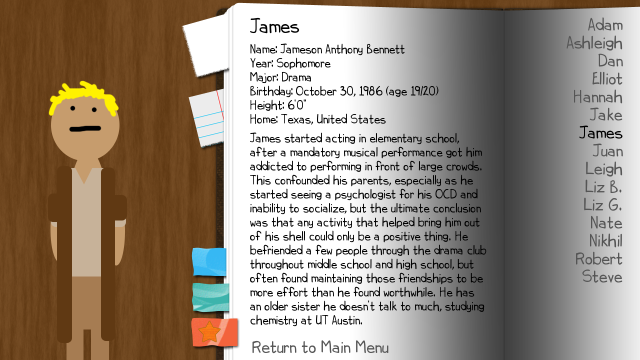
First of all, I defined a block of data for each character’s information. In addition, because these are linked from the title screen via a sketched sprite, I included coordinates for the appropriate sprite.
python early:
# list in reverse order of rendering on title screen
real_profile_names = (
"nick", "hannah", "elliot", "nate", "lizb", "lizg", "adam", "juan", "jake", "dan", "james",
)
profile_profile_info = {
"adam": ("Adam", (950,340),
"Sophomore", "Biology", "August 15, 1986 (age 20)", "6'2\"", "California, United States",
"Adam James Prewitt",
"Profile text for Adam"),
"juan": ("Juan", (850,340),
"Junior", "Biology", "April 22, 1986 (age 20)", "6'0\"", "Costa Rica",
"Juan Rodrigo Salas Valverde",
"Profile text for Juan"),
…etc…
}
Next we actually put these on the title screen.
screen main_menu():
…Stuff…
fixed:
if "completed" in persistent.profiles:
imagebutton auto "images/title/leigh_%s.png" action Show("char_profile", chname="ashleigh") xanchor 0.0 yanchor 0.0 pos (650, 140)
imagebutton auto "images/title/leigh_%s.png" action Show("char_profile", chname="leigh") xanchor 0.0 yanchor 0.0 pos (850, 140)
imagebutton auto "images/title/steve_%s.png" action Show("char_profile", chname="steve") xanchor 0.0 yanchor 0.0 pos (950, 140)
imagebutton auto "images/title/robert_%s.png" action Show("char_profile", chname="robert") xanchor 0.0 yanchor 0.0 pos (1050, 140)
for profname in real_profile_names:
if profname in persistent.profiles:
imagebutton auto "images/title/" + profname + "_%s.png" action Show("char_profile", chname=profname) xanchor 0.0 yanchor 0.0 pos profile_profile_info[profname][1]
Next, we define the screen for character profiles. Note the additional features that I’ll get into shortly.
screen char_profile(chname):
tag charprofile
xpos 0
ypos 0
default pmode = "normal"
fixed:
add "images/sp/profilebg.png" xpos 0 ypos 0
if pmode == "best":
add "images/title/profile_" + chname + "best.png" xalign 0.5 yalign 1.0 xpos 175 ypos 720
elif pmode == "good":
add "images/title/profile_" + chname + "good.png" xalign 0.5 yalign 1.0 xpos 175 ypos 720
else:
add "images/title/profile_" + chname + ".png" xalign 0.5 yalign 1.0 xpos 175 ypos 720
if (chname == "james" and persistent.permacc_james) or (…other guys’ checks…):
add "images/title/profile_" + chname + "acc.png" xalign 0.5 yalign 1.0 xpos 175 ypos 720
if chname + "best" in persistent.profiles or chname + "good" in persistent.profiles:
imagebutton auto "images/sp/proftab_normal_%s.png" action SetScreenVariable("pmode", "normal") xanchor 0.0 yanchor 0.0 pos (375, 631)
imagebutton auto "images/sp/proftab_good_%s.png" action SetScreenVariable("pmode", "good") xanchor 0.0 yanchor 0.0 pos (375, 559)
if chname + "best" in persistent.profiles:
imagebutton auto "images/sp/proftab_best_%s.png" action SetScreenVariable("pmode", "best") xanchor 0.0 yanchor 0.0 pos (375, 480)
imagebutton auto "images/sp/profextra_%s.png" action Start("epilogue_bonus_" + chname + "1") xanchor 0.0 yanchor 0.0 pos (350, 0)
imagebutton auto "images/sp/profpermacc_%s.png" action ToggleField(persistent, "permacc_" + chname) xanchor 0.0 yanchor 0.0 pos (363, 171)
vbox xpos 500 ypos 30 xalign 0.0 xsize 480:
spacing 10
text "{size=40}" + profile_profile_info[chname][0] + "{/size}"
text "{size=25}Name: " + profile_profile_info[chname][7] + "\nYear: " + profile_profile_info[chname][2] + "\nMajor: " + profile_profile_info[chname][3] + "\nBirthday: " + profile_profile_info[chname][4] + "\nHeight: " + profile_profile_info[chname][5] + "\nHome: " + profile_profile_info[chname][6] + "{/size}"
text "{size=25}" + profile_profile_info[chname][8] + "{/size}"
vbox xpos 1250 ypos 30 xalign 1.0 xsize 400:
for k in sorted(profile_profile_info):
if k in persistent.profiles or ("completed" in persistent.profiles and k in ("ashleigh", "leigh", "steve", "robert")):
textbutton profile_profile_info[k][0] action [Show("char_profile", chname=k), SelectedIf(chname == k)] xalign 1.0
textbutton "Return to Main Menu" action Hide("char_profile") xalign 0.0 xpos 500 ypos 675
As you can see, the profile screen has a few unlockable features. The first feature is buttons that let you view alternate poses for the character image, depending on which ending you’ve completed with the given person. This is implemented simply, with a screen variable indicating the mode.
The second feature is the ability to access a bonus scene for the given character, once you’ve found their HEA ending. Just define a label with the appropriate name (epilogue_bonus_james1 for example).
The last feature is a toggleable item on the guy that will persist on their sprite during subsequent playthroughs. For example, if you get James’ best ending, you can toggle his enagement ring on or off, and have it show up whenever he appears in the game. The associated code for that builds off of our sprite code and simply adds a new check for the persistent variable, and a layer.
image james jamesnew = LiveComposite(
(266, 700),
(0, 0), "chnew/james/[spposes_data[james].pose]/base.png",
(0, 0), "chnew/james/[spposes_data[james].pose]/basehair.png",
(0, 0), "chnew/james/[spposes_data[james].expression].png",
(0, 0), "chnew/james/[spposes_data[james].pants].png",
(0, 0), "chnew/james/[spposes_data[james].shirt].png",
(0, 0), "chnew/james/[spposes_data[james].permacc].png",
(0, 0), "chnew/james/[spposes_data[james].extras].png",
)
def execute_showsp(o):
…old code…
# Handle epilogue accessories
if who == "james" and persistent.permacc_james:
store.spposes_data[who].permacc = help_get_filepathsp(who, pose, "x", "ring")
…other guys' accessories…
else:
store.spposes_data[who].permacc = help_get_filepathsp(who, pose, "x", None)
…old code…
And… that’s it. Obviously, your profiles don’t have to be nearly this complicated. If you just want to show some basic information for each character, the screen and associated logic will be much simpler.